- About the Projects
- Prerequisites
- Planning the Projects
- Project: Navigation Cue Points in a .flv File
- Project: ActionScript Cue Points for Captions in an XML File
- Project: Basic Caption Display Template
- Project: Channeling Cue Points to the Caption Display
- Project: Code for Audio-only Captions
- Project: Advanced Captioning Template
- Project: Synchronized Images Template
- Exploring the Support Classes
- Final Thoughts
Project: Code for Audio-only Captions
To make a project like the one we just completed, but with audio this time, you use a nearly identical process. The only difference this time is that you can’t inject the .mp3 file with Navigation cue points. You can only use ActionScript cue points.
Steps
- Capturing the cue points
- Creating the main_audio.fla file
- Assembling support files
- Writing the code
- Alternative: using the MediaPlayback component
Step 1: Capturing the Cue Points
Follow the steps for the second project, "ActionScript Cue Points for Captions in XML File," but this time grab the file the_children_must_learn.mp3 from the source_media folder and the audio_captions.txt file from the starter_files folder. Enter those filenames when you run the gathering_tool.swf file, as shown in Figure 3.16.
When you’re done gathering cue points, be sure to click the Export XML button. Then copy and paste the XML string into a text file you save as audio_captions.xml.
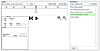
Figure 3.16 You’ll use the same gathering_tool.swf but this time for an audio track only.
Step 2: Creating the main_audio.fla File
Create a new Flash file and save it in your working directory as main_audio.fla. Either copy an instance of the CaptionHolder symbol created in the last project, "Cue Points in the Caption Display," or repeat step 1 of the preceding project.
Place on stage an instance of CaptionHolder and give it an instance name of captions_clip (just like before).
Step 3: Assembling Support Files
Ensure that your working directory has, at least, the following support files in addition to main_audio.fla (you’ll find the .as files in the starter_files folder and the .mp3 in the starter_media folder—you need to create the .swf and .xml files yourself):
- the_children_must_learn.mp3
- audio_captions.xml
- captionType1.swf
- CaptionHolder.as
- EventChannel.as
Step 4: Writing the Code
In the main_audio.fla file, select the first keyframe and open the Actions panel. Type the code shown in Listing 3.3.
Listing 3.3 This Code Channels Captions for an Audio Track to the Same captionType1.swf Used Earlier
1 var myEventChannel:EventChannel = new EventChannel(); 2 3 var url = "captionType1.swf"; 4 var eventList = ["actionscript"]; 5 captions_clip.init( url, myEventChannel, eventList ); 6 7 var mySound:Sound = new Sound(); 8 9 function ready(){ 10 mySound.loadSound( "the_children_must_learn.mp3", true ); 11 } 12 myEventChannel.addEventListener( "ready", this ); 13 14 myEventChannel.init( mySound, "audio_captions.xml" );
In Listing 3.3, I was extra careful not to start the audio until the ready event fires. If you don’t have any captions at the start of the sound, you can safely start playing the sound anytime you want and forgo lines 9–12. The only critical sequence issues are that myEventChannel is instantiated before you invoke init() on the captions_clip (because you’re passing a reference to the myEventChannel instance) and that the mySound instance is instantiated before you invoke init() on myEventChannel (because you’re passing a reference to the mySound instance).
Save and test the movie.
Step 5: Alternative: Using the MediaPlayback Component
Before I show you how to use the MediaPlayback component for this audio-only project, I want to tell you about the two advantages it offers. The first benefit is that it works with .mp3 audio files as well as .flv videos. Second, because the FLVPlayback component requires Flash Player 8, you’ll want to use the MediaPlayback component if you’re delivering a project to Flash Player 6 or 7.
Using the MediaPlayback component instead of the FLVPlayback component is simple. Open the Components panel and drag a MediaPlayback instance onstage (from the Media - Player 6-7 category). Give it an instance name of myMediaPlayback. Replace the code you wrote in step 4 with the code in Listing 3.4.
Basically, you can pass an FLVPlayback instance, a Sound instance, or—as shown here—a MediaPlayback component instance. The cool part about this version shown in Figure 3.17 is that, because you’re using the MediaPlayback component, you automatically get a bunch of features, such as pause, play, and scrubbing. Remember that, although the FLVPlayback component also supports these features, it works only with video.
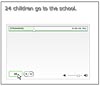
Figure 3.17 The MediaPlayback component is hard to resist when playing audio because it includes playback controls and is easy to use.
Listing 3.4 This Code Plays a .mp3 (and Its Captions) Through the MediaPlayback Component
import mx.controls.MediaPlayback; var myMediaPlayback:MediaPlayback; myMediaPlayback.contentPath = "the_children_must_learn.mp3"; var myEventChannel:EventChannel = new EventChannel(); var url = "captionType1.swf"; var eventList = ["actionscript"]; captions_clip.init(url, myEventChannel, eventList); myEventChannel.init(myMediaPlayback, "audio_captions.xml");