Tools of the Trade, Part 2: Building Graphs with JGraph
For more information on Java development, visit our Java Reference Guide or sign up for our Java Newsletter.
Last time, I introduced you to a series on useful open-source tools that could prove beneficial to your Java career. The inaugural article focused on iText, an open-source Java library for creating PDF, HTML, RTF, and XML documents. This article continues the series by presenting JGraph.
Computer science defines a graph as a nonempty finite set of vertices (labelled points) and a set of edges (pairs of vertices) that signify connections between the vertices. Graphs represent networks of related and (typically) interconnected objects such as roads, molecules, database schemas, and the Internet. Although the graph concept is easy to understand, creating the software to construct, display, and manipulate graphs can be difficult. Thanks to Java and a utility called JGraph, you do not need to create your own graph software.
This article introduces you to JGraph. After showing you how to acquire JGraph, install this tool, and set the CLASSPATH environment variable, I present a simple Java application that uses JGraph to create and display a sample graph. This application introduces you to several operations that JGraph provides for graph manipulation. The article then explores several important areas of JGraph’s architecture. This JGraph overview provides you with enough knowledge to quickly start creating your own graphs.
JGraph Intro
JGraph, created by Gaudenz Alder, is an open-source, Swing-based component for building, displaying, and manipulating graphs. JGraph is available as a free version under the GNU Lesser General Public License (LGPL) and as a commercial version under the JGraph General License. The main difference between these licenses: LGPL requires you to include JGraph’s source code (including any modifications you make to JGraph) when distributing an application that uses JGraph; JGraph’s source code does not need to be included under the General License.
Visit the JGraph Free Downloads page to download the binary and source distributions for the latest free version of JGraph. You need only the binary distribution for this article: Click the Download Latest Binary Distribution link to obtain that distribution. After the download finishes, you will discover jgraph-latest-lgpl.jar on your hard drive.
From the command line, invoke java -jar jgraph-latest-lgpl.jar to install JGraph. After agreeing to all terms in the license agreement, choose an installation directory, such as c:\jgraph (which is the installation directory assumed in this article). JGraph installs to this directory. If installation is successful, the installation directory will contain the following files and directories:
- ChangeLog identifies those changes introduced by each version of JGraph.
- LICENSE presents version 2.1 of the LGPL.
- README introduces JGraph and provides instructions for building this tool from its source code.
- WHATSNEW describes new features and bug fixes introduced by each JGraph version. This file’s content is similar to the content found in ChangeLog.
- doc provides JGraph documentation. Its api subdirectory contains Javadoc files that describe the API. The pdf subdirectory contains an updating.pdf file with information that can help you update existing JGraph code to the latest version.
- examples provides a package of Java programs that demonstrate JGraph features.
- lib provides the jgraph.jar Jar file, which you must add to your CLASSPATH environment variable before you can use JGraph. For Windows 98 SE, the command to set CLASSPATH is set classpath=%classpath%;c:\jgraph\lib\jgraph.jar;. If you are using a different version of Windows, or a non-Windows operating system, study your OS documentation to find out how to set this environment variable.
Now that JGraph has been downloaded, installed, and configured, we can explore a Java application that uses JGraph to create a sample graph. Take a look at Listing 1.
Listing 1 SampleGraph.java
// SampleGraph.java import org.jgraph.JGraph; import javax.swing.*; public class SampleGraph extends JFrame { public SampleGraph (String title) { super (title); setDefaultCloseOperation (EXIT_ON_CLOSE); getContentPane ().add (new JScrollPane (new JGraph ())); pack (); setVisible (true); } public static void main (String [] args) { new SampleGraph ("Sample Graph"); } }
Listing 1 describes a Swing application that introduces a sample graph into its GUI. The application’s constructor introduces the sample graph by creating an org.jgraph.JGraph object and invoking its public JGraph() constructor to initialize this object. The JGraph object is then placed into a JScrollPane (to make sure that the entire graph is viewable, even when the graph exceeds its display area because the window has been resized smaller) and the JScrollPane is added to the JFrame’s content pane.
After compiling Listing 1, run this application. You will see the sample graph that is presented in Figure 1. The rectangles serve as the graph’s vertices, and the lines serve as its edges.
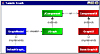
Figure 1 The sample graph reveals JGraph component architecture.
Figure 1’s sample graph is not just for show: You can also manipulate this graph by performing various operations. For example, double-click the vertex that displays JComponent (or single-click that vertex to select it and then press F2). In response, an editor appears over the vertex to perform in-place editing of the vertex’s label. Type abc into that text field and then press Enter. You should now see abc instead of JComponent. Figure 2 reveals the editor and the entered label.

Figure 2 You can perform in-place editing on a vertex or an edge.
When you select a vertex, you will notice small handles (rectangles that you can grip for sizing) in the vertex’s corners and along its sides. Similarly, the selection of an edge results in handles at the source and target of that edge. These handles make it possible to resize the vertex or edge. Accomplish that task by using the mouse to select and drag a handle: The vertex or edge resizes in that direction. For example, resize the JGraph vertex by selecting that vertex, gripping the handle in the lower-right corner, and dragging the handle downward and to the right. Figure 3 shows this vertex expanding.

Figure 3 A vertex changes color during a resize operation.
You can select a combination of vertices and edges by holding down Shift during the selection process. If you accidentally select a vertex or edge that you do not want to be included, release Shift and press Ctrl to deselect that vertex/edge. A pair of selected vertices and the edge that connects those vertices are dragged to another location in Figure 4.

Figure 4 An edge between two selected vertices is automatically selected.
Resizing or dragging a selection—you can abort either operation by pressing Esc—is accomplished through a cell handle. In contrast to an editor that uses a text component to change a vertex/edge label through in-place editing, a cell handle uses other means (such as performing a color change and showing grayed edges in their new locations) to provide users with visual feedback on how a graph will look after the change has been made. JGraph refers to this feature as live-preview.
JGraph supports the placement of related vertices and edges into groups, a mechanism for structurally organizing a graph. The presence of groups within a graph affects the selection process. When selecting a vertex or edge within a group, the first mouse click on the vertex or edge selects the group (whose members can then be resized or dragged as a single unit—you can even edit the group’s name); the second mouse click selects the vertex/edge. (If groups are nested within other groups, it can take multiple clicks on the vertex or edge before that vertex/edge is selected.) Figure 5 illustrates this stepping into groups feature. Notice the dashed outline around a group, consisting of vertex GraphModel, edge implements, and vertex DefaultGraphModel. That outline indicates the selection of the group. Also notice the selection of the implements edge, whose label appears in the editor.

Figure 5 Click once for the group and twice for the edge.
You can select multiple groups, vertices, and edges by pressing Shift and clicking each entity, but that is somewhat cumbersome. If those entities exist within a rectangular area, an easier way to accomplish this task involves marquee selection.
Marquee selection is a selection mechanism in which you move the mouse pointer to any location in the graph and press a mouse button. As you drag the mouse pointer, you observe a rectangle—known as a marquee—that expands or contracts, based on the direction of movement. When you release the mouse button, the marquee disappears; all groups, vertices, and edges completely within the marquee are selected. Figure 6 reveals the marquee. When the mouse button is released, the JComponent vertex, the extends edge, and the JGraph vertex will be selected.
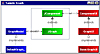
Figure 6 Select a group by including all vertices and edges within the marquee.
Sometimes, you will want to more accurately place a vertex, an edge, or a group during a drag operation—by constraining the drag to horizontal and vertical movement. In other words, you do not want to drag diagonally. JGraph provides a constrained drag operation to help you accomplish this task. That operation requires you to hold down Shift while dragging the mouse.
There is one final operation to consider: clone selection (the duplication of a vertex, an edge label, or a group by holding down the Ctrl key while dragging the mouse). Unlike constrained drag, clone selection cannot be demonstrated in SampleGraph without changing the source code. The change requires replacing the following line of code:
getContentPane ().add (new JScrollPane (new JGraph ()));
with this code fragment:
graph = new JGraph (); graph.setCloneable (true); getContentPane ().add (new JScrollPane (graph));
Modify SampleGraph.java to incorporate the code fragment above, compile the source code, and run the application. Position the mouse pointer over a vertex, an edge label, or a group member and then hold down Ctrl while dragging the mouse. You will end up with a clone of the vertex, edge label, or group.
For more practice with JGraph’s operations, you will want to check out the graph editor example that ships with JGraph. Compile the graph editor’s source code, which resides in c:\jgraph\examples\org\jgraph\example\GraphEd.java, and run that program as an application—you can also run the graph editor as an applet. Figure 7 reveals the graph editor’s GUI.
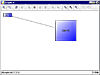
Figure 7 The graph editor lets you create, drag, and connect vertices.
The graph editor’s toolbar presents several icons. Click the leftmost icon to introduce a vertex into the GUI. The icon immediately to the right, when it appears as an arrow, causes a small rectangle to appear in the midst of a vertex. Selecting that rectangle and dragging the mouse results in an edge being drawn from the vertex (presumably you want to draw the edge to another vertex). But if the icon appears with a stylized red X drawn on top, you cannot draw an edge.