Understanding the App Package
The package manifest in the Visual Studio project is a file called Package.appxmanifest. (“AppX” is a term sometimes used within Microsoft for Windows Store app packages that stuck around in the filename.) This manifest describes your app to Windows as well as the Windows Store—its name, what it looks like, what it’s allowed to do, and more. It’s an XML file, although you have to tell Visual Studio to “View Source” in order to see the XML. There’s usually no need to view and edit the XML directly, however. The default view is a tabbed set of forms to fill out, which is the easiest way to populate all the information. There are six tabs:
- Application
- Visual Assets
- Capabilities
- Declarations
- Content URIs
- Packaging
For our HelloRealWorld app, we don’t need to change anything in the package manifest. But now is a good time to understand what can be done on each of these tabs.
Application
On the Application tab, you can set the app’s name and description, default language, its minimum width, and notification settings (if your app supports them). Notifications are covered in Chapter 25, “Thinking Outside the App: Live Tiles, Notifications, and the Lock Screen.” You can even restrict the preferred orientations of your app if you’d rather not have it automatically rotate to all four of them:
- Landscape (horizontal)
- Landscape-flipped (horizontal but upside down)
- Portrait (vertical, with the hardware Start button on the left)
- Portrait-flipped (vertical, with the hardware Start button on the right)
Disabling the flipped orientations would be an odd thing to do, but disabling some orientations can make sense for certain types of games that wish to be landscape only. Note that this is just a preference, not a guarantee, because not all devices support rotation. For example, a portrait-only app launched on a typical desktop PC must accept the one-and-only landscape orientation. However, if a device that does support rotation is currently locked to a landscape orientation, a portrait-only app actually runs in the portrait orientation, ignoring the lock setting.
Visual Assets
On the Visual Assets tab, you set the characteristics of your app’s tile and splash screen, as well as artwork used in a number of other contexts.
Customizing the Splash Screen
To ensure that every app’s splash screen can be displayed practically instantaneously (before your app even gets loaded), you have little control over it. You specify a 620x300 image (plus two optional larger sizes to support high DPI screens), and a background color for the splash screen. That’s it. Visual Studio gives you an appropriately sized placeholder SplashScreen.scale-100.png file in an Assets subfolder, intentionally made ugly to practically guarantee you won’t forget to change it before submitting your app to the Windows Store.
When your splash screen is shown, the image is displayed centered on top of your chosen background color. Figure 1.3 shows an example SplashScreen.scale-100.png containing a Pixelwinks logo, and Figure 1.4 shows what this looks like on the simulator. The splash screen is given a yellow background for demonstration purposes. A real app should make the background color match the background of the image or simply make the image’s background transparent.
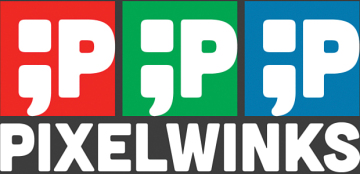
FIGURE 1.3 An example SplashScreen.scale-100.png with a nontransparent background for demonstration purposes

FIGURE 1.4 A live splash screen shown inside the simulator with a garish yellow background to clearly show the bounds of the image
When your app is launched, the splash screen automatically animates in and automatically fades out once your app has loaded and has made a call to Window.Current.Activate. This gives you the flexibility to do arbitrarily complex logic before the splash screen goes away, although you should avoid doing a lot of work here. (Your app is given about fifteen seconds to remain on the splash screen before it gets terminated by Windows.)
Customizing Logo Images
The Tile Images and Logos section on the Visual Assets tab can be confusing and overwhelming. Besides the Store Logo, which supports up to three different sizes, it lists five different logo sizes, although each one actually accepts 4–8 different sizes of image files! All told, you can assign twenty seven different image files representing your logo! Let’s start making some sense out of these images. Figure 1.5 shows what each logo should have been called to make things less confusing, and the following list explains each one using the terminology found in the package manifest:
- Square 70x70 Logo—This is used for the small version of your app’s tile on the Start screen. Although assigning an image here is optional, the small tile size is not. If you don’t provide an image, the medium tile image is used (and scaled down) when a user changes your tile size to small.
- Square 150x150 Logo—This is used for the medium version of your app’s tile on the Start screen. The medium tile size is the one required size, so at least a 100% scale image is required.
- Wide 310x150 Logo—This is used for the wide version of your app’s tile on the Start screen, if you choose to support that tile size. If you assign at least a 100% scale image here, your app automatically supports the wide tile size. Otherwise, it doesn’t.
- Large 310x310 Logo—This is used for the large version of your app’s tile on the Start screen, if you choose to support that tile size. If you assign at least a 100% scale image here and for the wide logo, your app automatically supports the large tile size. (Your app can only support a large tile if it also supports a wide tile.) Otherwise, it doesn’t.
- Square 30x30 Logo—This is used throughout Windows, including on the desktop. It is used by the apps list, search results, the Share pane, the file picker, an overlay on live tiles, the Alt+Tab user interface, Task Manager, file icons for associated file types, and so on. At least the 100% scale image is required. Although the image is nominally 30x30 pixels, this logo supports four additional sizes to be used for file icons on the desktop (if your app has associated file types): 16x16, 32x32, 48x48, and 256x256.
- Store Logo—A 50x50 image (at 100% scale) used by the Windows Store. At least the 100% scale image is required.
Visual Studio provides placeholder image files for the required logo images only: the square 150x150 logo, the square 30x30 logo, and the store logo.

FIGURE 1.5 More understandable names for the different logo images you can provide
As with the splash screen, you can specify a background color for your tile. For the best results, this color (as well as the tile images) should match what you use in your splash screen. The desired effect of the splash screen is that your tile springs to life and fills the screen in a larger form. Even if your tile background color is completely covered by opaque tile images, there are still contexts in which the color is seen, such as the zoomed-out Start screen view or the Alt+Tab user interface. Therefore, choose your background color (and determine whether you want your images to use transparency) carefully!
You can choose a “default size,” which is the initial size of your tile if the user decides to pin it to the Start screen. This can only be set to the medium tile or the wide tile (if you support a wide tile). If unset, wide is given precedence over medium.
You can also choose a “short name,” which is the text that gets overlaid on the bottom of your tile. You can even specify which tile sizes should show the text: medium, wide, and/or large. (Small tiles do not support overlaid text.) Many apps turn off the text because their images already include a logo with the name.
Finally, you can decide whether you want the overlaid text to be “light” (which means white) or “dark” (which means a dark gray). Although most apps use white text, you may need to choose the dark option if you want your tile to have a light background color.
Capabilities
On the Capabilities tab, you select each capability required by your app. A capability is a special permission for actions that users might not want certain apps to perform, whether for privacy concerns or concerns about data usage charges. In the Windows Store, prospective users are told what capabilities each app requires before they decide whether to download it. To users, they are described as permissions, sometimes with more descriptive names, as shown in Figure 1.6.

FIGURE 1.6 The Fresh Paint app uses three capabilities: Pictures Library, Webcam, and Internet (Client).
For the most part, user approval of all requested permissions is an implicit part of downloading an app. However, the use of privacy-related capabilities, such as location services, prompts the user the first time an app invokes a relevant API. Furthermore, some capabilities can be disabled or reenabled at any time by a user. When the Settings charm is invoked while a Windows Store app is running, it contains a “Permissions” link that displays an app’s capabilities and toggle switches for any that can be turned on and off. Figure 1.7 shows what this looks like while running HelloRealWorld, both with the default capability already chosen in our package manifest—Internet (Client)—and after selecting every listed capability in the package manifest.

FIGURE 1.7 The “Permissions” section of the Settings charm lists the current app’s capabilities, and enables turning some of them on or off at runtime.
The long list of available capabilities can be grouped into four different categories:
- File capabilities
- Device capabilities
- Network capabilities
- Identity capabilities
Most of them can be used freely, although some of them are restricted. Apps that use restricted capabilities must go through extra processes when uploaded to the Windows Store and are only granted to business developer accounts with written justification. Fortunately, the restricted capabilities (called out in the upcoming lists) are for uncommon scenarios.
File Capabilities
As you’ll read in Chapter 20, “Working with Data,” apps can read and write their own private files in an isolated spot, and those files can even participate in automatic roaming between a user’s devices. In addition, users can give apps explicit permission to read/write other “normal” files and folders via the Windows file picker. This is all that most apps need, and does not require any capabilities.
Beyond these two features, however, programmatic reading and writing of files requires special capabilities. There is one for each of the four built-in libraries (Documents, Music, Pictures, and Videos) plus another for attached storage devices:
- Music Library, Pictures Library, and Videos Library—Enables enumerating and accessing all music, pictures, and videos, respectively, without going through the file picker.
- Documents Library—Enables adding, changing, and deleting files in the Documents library on the local computer without going through the file picker. However, this capability is restricted to specific file type associations that must also be declared in the package manifest (on the Declarations tab). This is listed separately from the preceding three capabilities because it is a restricted capability that needs special approval from Microsoft in order to publish the app in the Windows Store. And unlike the capabilities for the Music, Pictures, and Videos libraries, this cannot be used to access Documents libraries on other computers in the same HomeGroup.
- Removable Storage—Enables adding, changing, and deleting files on devices such as external hard drives or thumb drives connected to the local computer, again without going through the file picker. As with the preceding capability, this is restricted to file type associations that must also be declared in the package manifest.
Device Capabilities
Apps can access simple sensors such as an accelerometer or devices such as a printer without any capabilities. Accessing other sensors or devices does require specific capabilities, however. The list of device types grows over time (and can be extended by third parties), but the Capabilities tab exposes four choices, listed below. For all of them except proximity, users can disable them at any time, so apps must be prepared to handle this gracefully.
- Location—Reveals the computer’s location, either precise coordinates from a GPS sensor (if one exists) or an estimation based on network information.
- Microphone—Enables recording audio from a microphone.
- Webcam—Enables recording video—or capturing still pictures—from a camera. Note that this doesn’t include sound. If you want to record audio and video, you need both Webcam and Microphone capabilities.
- Proximity—Enables communication with nearby devices, either via Wi-Fi Direct or near field communication (NFC).
Chapters 14, “Audio, Video, and Speech,” and 23, “Reading from Sensors,” explain how to write apps that take advantage of these capabilities. Additional device capabilities exist that don’t appear on the Capabilities tab. These must be added manually to the package manifest XML. See Chapter 24, “Controlling Devices,” for more information.
Network Capabilities
Without any network capabilities, a Windows Store app cannot do any communication over any kind of network except for the automatic roaming of application data described in Chapter 20, the seamless opening/saving of network files enabled by the file picker, or the peer-to-peer connections enabled by the Proximity capability. Four types of network capabilities exist:
- Internet (Client)—This is the only network capability that most apps need. It provides outbound access to the Internet and public networks (going through the firewall).
- Internet (Client & Server)—This is just like the preceding capability except it provides both inbound and outbound access, which is vital for peer-to-peer apps. It’s a superset of “Internet (Client)” so if you request this capability in your manifest, then you don’t need to request the other one.
- Private Networks (Client & Server)—Provides inbound and outbound access to trusted home and work networks (going through the firewall).
- Enterprise Authentication—Enables intranet access using the current Windows domain credentials. This is a restricted capability.
Identity Capabilities
This is not really a fourth category of capabilities, but rather a single outlier that doesn’t fit anywhere else. The Shared User Certificates capability enables access to digital certificates that validate a user’s identity. The certificate could be installed on the computer or stored on a smart card. This is mainly for enterprise environments, and it is a restricted capability.
Declarations
The Declarations tab is the one with the most options. This is where you declare your app’s support for one or more contracts, if applicable. Contracts enable your app to cooperate with another app, or Windows itself, to complete a well-defined task. Every contract has a source that initiates the task and a target that completes it.
Your app can be the source for a contract without doing anything in the package manifest. (It just makes various API calls.) To be the target, however, your app must be activated in a special manner. This is what requires the declaration in the package manifest. Therefore, you can think of the list of available declarations as the list of available contract targets.
Unlike capabilities, contract target declarations are not listed in the Windows Store as potentially unwanted features. In fact, you should go out of your way to mention your supported contract scenarios, because they can be very useful! There’s nothing about being a contract target that is inherently dangerous for the user. Supporting certain contracts does require relevant capabilities, but many don’t require any. See Chapter 22, “Leveraging Contracts,” for specific examples.
Content URIs
This tab, new to Windows 8.1, only applies if you are hosting HTML content inside your XAML app. It simply houses a list of HTTPS URLs whose JavaScript is allowed (or disallowed) to raise events that can be handled by your app. For more information, see the discussion of the WebView control in Chapter 15, “Other Controls.”
Packaging
The Packaging tab is meant to describe information needed for the app’s listing in the Windows Store. However, for apps in the store, this information is managed by the Windows Dev Center dashboard. You therefore don’t normally need to change these values in your local package manifest:
- The package name is a unique identifier. Visual Studio automatically fills it in with a globally-unique identifier known as a GUID. That said, for easier debugging and identification of your app’s local data store, it’s best to replace the GUID with a human-readable name, such as CompanyName.AppName. This name doesn’t impact real users of your app, as the Windows Store assigns this value in the package that users download.
- The package display name is the name of your app in the store, but this also gets replaced when you follow the procedure to upload an app, so you can leave this item alone.
The version, set to 1.0.0.0 by default, is a four-part value interpreted as Major.Minor.Build.Revision. You can set this value however you like. There are only two requirements enforced by the Windows Store:
- Each new published version has a higher version number than previous published versions (for the same target version of Windows).
- If your app simultaneously has a package for Windows 8 and a package for Windows 8.1, the Windows 8 package version number must never exceed the version number of your first published Windows 8.1 package.
- The bottom of this tab contains publisher information based on the certificate used to authenticate the package. Visual Studio configures this to work with the temporary certificate it generates, and the store upload process reconfigures it to work with your developer account.
For testing certain notification or purchase scenarios that depend on an app’s identity in the Windows Store, you can automatically update your local package manifest’s packaging values to match the values maintained by the Windows Store. To do this, you can select Associate App with the Store..., which can be found on the Store menu in Visual Studio Express or on the Project, Store menu in other editions.