- 4.1 Introduction
- 4.2 Algorithms
- 4.3 Pseudocode
- 4.4 Control Structures
- 4.5 If...Then Selection Statement
- 4.6 If...Then...Else Selection Statement
- 4.7 Nested If...Then...Else Statements
- 4.8 Repetition Statements
- 4.9 Compound Assignment Operators
- 4.10 Formulating Algorithms: Counter-Controlled Repetition
- 4.11 Formulating Algorithms: Nested Control Statements
- 4.12 Using the Debugger: Locating a Logic Error
- 4.13 Wrap-Up
- Summary
- Terminology
- Self-Review Exercises
- Answers to Self-Review Exercises
- Quick Quiz
- Exercises
- Making a Difference Exercises
4.11 Formulating Algorithms: Nested Control Statements
We've seen that control statements can be stacked on top of one another (in sequence). We now examine the only other structured way that control statements can be combined—by nesting one control statement inside another.
Problem Statement and Notes
Consider the following problem statement:
- A college offers a course that prepares students for the state real estate broker licensing exam. Last year, 10 of the students who completed this course took the exam. The college wants to know how well its students did. You've been asked to write a program to summarize the results. You've been given a list of the 10 students. Next to each name is written a "P" if the student passed the exam and an "F" if the student failed the exam.
- Your program should analyze the results of the exam as follows:
- Input each exam result (that is, a "P" or an "F").
- Count the number of passes and the number of failures.
- Display a summary of the exam results, indicating the number of students who passed and the number who failed.
- If more than eight students passed the exam, display the message "Bonus to Instructor."
After reading the problem statement carefully, we make the following observations:
- The program must process exam results for 10 students. A counter-controlled loop can be used because the number of test results is known in advance.
- Each exam result is a string—either a "P" or an "F." When the program reads an exam result, it must determine whether the result is a "P" or an "F." We test for a "P" in our algorithm. If the input is not a "P," we assume it's an "F." An exercise at the end of the chapter considers the consequences of this assumption. For instance, consider what happens when the user enters a lowercase "p."
- Two counters store the exam results—one counts the number of students who passed the exam and the other counts the number of students who failed.
- After the program has processed all the exam results, it must determine whether more than eight students passed the exam, and, if so, bonus the instructor.
GUI for the Licensing-Exam Analysis Application
Figure 4.13 shows this application's GUI. Each result is placed in the ListBox when the user presses the Submit Result Button. We process all 10 results when the user presses the Analyze Results Button. We also provide a Clear Results Button to reset the GUI so the user can enter a new set of results. As in the previous example, we used the Form's Accept-Button property to set the Submit Result Button as the default Button. We disabled the Analyze Results Button initially by setting its Enabled property to False.
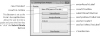
Fig. 4.13 GUI for the licensing-exam analysis problem.
Developing the Pseudocode Algorithm with Top-Down, Stepwise Refinement: The Top and the First Refinement
We approach this program with top-down, stepwise refinement, a technique for developing well-structured algorithms. We begin with a pseudocode representation of the top—a single statement that conveys the overall function of the program:
Analyze exam results and decide if the instructor should receive a bonus
The top is a complete representation of a program. Unfortunately, the top rarely conveys enough detail from which to write a program, so we conduct the refinement process. We divide the top into a series of smaller tasks and list them in the order in which they must be performed, resulting in the following first refinement:
Initialize variables Input the 10 exam results, and count passes and failures Display a summary of the exam results and decide if the instructor should receive a bonus
Proceeding to the Second Refinement
To proceed to the second refinement, we commit to specific variables. In this example, counters are needed to record the passes and failures. A counter controls the looping process and a variable stores the user input. The pseudocode statement
Initialize variables
can be refined as follows:
Initialize passes to zero Initialize failures to zero Initialize student to zero
Only the counters for the number of passes, number of failures and number of students need to be initialized before they're used. The variable we use to store each value the user enters does not need to be initialized because the assignment of its value does not depend on its previous value, as is the case for the counter variables.
The pseudocode statement
Input the 10 exam results, and count passes and failures
requires a repetition statement (that is, a loop) that successively inputs the result of each exam. We know in advance that there are 10 exam results, so counter-controlled looping is appropriate. Inside the loop (that is, nested inside the loop), a double-selection statement will determine whether each exam result is a pass or a failure and will increment the appropriate counter. The refinement of the preceding pseudocode statement is then
While student counter is less than or equal to 10 Get the next exam result If the student passed then Add one to passes Else Add one to failures Add one to student counter
Note the use of blank lines to set off the If...Else control statement to improve readability.
The pseudocode statement
Display a summary of the exam results and decide if the instructor should receive a bonus
may be refined as follows:
Display the number of passes Display the number of failures If more than eight students passed then Display "Bonus to instructor!"
Complete Second Refinement of Pseudocode
The complete second refinement of the pseudocode appears in Fig. 4.14. Blank lines are also used to set off the While loop (lines 5–13) for readability. This pseudocode is now sufficiently refined for conversion to Visual Basic.
Fig 4.14. Pseudocode for examination-results problem.
1 Initialize passes to zero 2 Initialize failures to zero 3 Initialize student to zero 4 5 While student counter is less than or equal to 10 6 Get the next exam result 7 8 If the student passed then 9 Add one to passes 10 Else 11 Add one to failures 12 13 Add one to student counter 14 15 Display the number of passes 16 Display the number of failures 17 18 If more than eight students passed then 19 Display "Bonus to instructor!" |
Class Analysis
The program that implements the pseudocode algorithm and the sample outputs are shown in Fig. 4.15. We defined the Click event handlers for the submitResultButton, analyzeResultsButton and clearResultsButton. The analyzeResultsButton_Click method (lines 24–55) implements the class-averaging algorithm described by the pseudo-code in Fig. 4.14.
Fig 4.15. Nested control statements: Examination-results problem.
1 ' Fig. 4.15: Analysis.vb 2 ' Nested control statements: Examination-results problem. 3 Public Class Analysis 4 ' place a result in the resultsListBox 5 Private Sub submitResultButton_Click(ByVal sender As System.Object, 6 ByVal e As System.EventArgs) Handles submitResultButton.Click 7 8 If resultsListBox.Items.Count < 10 Then 9 ' add the grade to the end of the resultsListbox 10 resultsListBox.Items.Add(resultTextBox.Text) 11 resultTextBox.Clear() ' clear the resultTextBox 12 resultTextBox.Focus() ' select the resultTextBox 13 End If 14 15 ' determine whether to prevent the user from entering more results 16 If resultsListBox.Items.Count = 10 Then 17 submitResultButton.Enabled = False ' disables submitResultButton 18 resultTextBox.Enabled = False ' disables resultTextBox 19 analyzeResultsButton.Enabled = True ' enable analyzeResultsButton 20 End If 21 End Sub ' submitResultButton_Click 22 23 ' analyze the results 24 Private Sub analyzeResultsButton_Click(ByVal sender As System.Object, 25 ByVal e As System.EventArgs) Handles analyzeResultsButton.Click 26 27 ' initializing variables in declarations 28 Dim passes As Integer = 0 ' number of passes 29 Dim failures As Integer = 0 ' number of failures 30 Dim student As Integer = 0 ' student counter 31 Dim result As String ' one exam result 32 33 ' process 10 students using counter-controlled loop 34 Do While student < 10 35 result = resultsListBox.Items(student) ' get a result 36 37 ' nested control statement 38 If result = "P" Then 39 passes += 1 ' increment number of passes 40 Else 41 failures += 1 ' increment number of failures 42 End If 43 44 student += 1 ' increment student counter 45 Loop 46 47 ' display exam results 48 analysisResultsLabel.Text = 49 "Passed: " & passes & vbCrLf & "Failed: " & failures & vbCrLf 50 51 ' raise tuition if more than 8 students passed 52 If passes > 8 Then 53 analysisResultsLabel.Text &= "Bonus to instructor!" 54 End If 55 End Sub ' analyzeResultsButton_Click 56 57 ' clears the resultsListBox and analysisResultsLabel 58 Private Sub clearResultsButton_Click(ByVal sender As System.Object, 59 ByVal e As System.EventArgs) Handles clearResultsButton.Click 60 61 resultsListBox.Items.Clear() ' removes all items 62 analysisResultsLabel.Text = String.Empty ' clears the text 63 submitResultButton.Enabled = True ' enables submitResultButton 64 resultTextBox.Enabled = True ' enables resultTextBox 65 analyzeResultsButton.Enabled = False ' disables analyzeResultsButton 66 resultTextBox.Focus() ' select the resultTextBox 67 End Sub ' clearResultsButton_Click 68 End Class ' Analysis |
Method submitResultButton_Click
When the user presses the Submit Result button, method submitResultButton_Click (lines 5–21) executes. The problem statement specified that the user can enter only 10 results, so we need to keep track of the number of results that have been entered. Since the ListBox property Items keeps track of the ListBox's total number of items, we use its Count property in line 8 to determine whether 10 results have been entered. If not, line 10 adds the next result to the ListBox, line 11 clears the TextBox and line 12 gives the Text-Box the focus so the user can enter the next result. The last result entered could have been the 10th result (line 16). If so, lines 17–18 disable the submitResultButton and the resultTextBox by setting their Enabled properties to False. This prevents the user from interacting with these two controls, so the user cannot enter more than 10 results. Line 19 enables analyzeResultsButton by setting its Enabled property to True so the user can tell the program to analyze the results.
Method analyzeResultsButton_Click
Method analyzeResultsButton_Click (lines 24–55) uses the algorithm in Fig. 4.14 to calculate the class average and display the results. Lines 28–31 declare the variables the algorithm uses to process the examination results. Several of these declarations incorporate variable initialization—passes, failures and student are all initialized to 0.
The Do While...Loop statement (lines 34–45) loops 10 times. During each iteration, the loop inputs and processes one exam result from the ListBox. The If...Then...Else statement (lines 38–42) for processing each result is nested in the Do While...Loop statement. If the result is "P", the If...Then...Else statement increments passes; otherwise, it assumes the result is "F" and increments failures. Strings are case sensitive by default—uppercase and lowercase letters are different. Only "P" represents a passing grade. In Exercise 4.13, we ask you to enhance the program by processing lowercase inputs such as "p" and "f".
Line 44 increments student before the loop condition is tested again at line 34. After 10 values have been processed, the loop terminates and lines 48–49 display the number of passes and failures. The If...Then statement at lines 52–54 determines whether more than eight students passed the exam and, if so, displays "Bonus to instructor!". In the first sample execution, the condition at line 52 is True—more than eight students passed the exam, so the program outputs the bonus message.
Method clearResultsButton_Click
When the user presses the Clear Results Button, method clearResultsButton_Click (lines 58–67) prepares for the user to enter a new set of results. Line 61 removes the results from the resultsListBox by calling the Item property's Clear method. Line 62 clears the text on the analysisResultsLabel. Lines 63 and 64 enable the submitResultButton and the resultTextBox by setting their Enabled properties to True—the user can now interact with these controls again. Line 65 disables the analyzeResultsButton by settingits Enabled property to False so the user cannot click it. Line 66 gives the focus to the result-TextBox so the user can begin typing the next result immediately.