Allow users to sign up on your website, from collecting signup information with an HTML form and rendering a profile page, to validating users by adding a separate account activation step.
Now that we have a working User model, it’s time to add an ability few websites can live without: letting users sign up. We’ll use an HTML form to submit user signup information to our application (Section 7.2), which will then be used to create a new user and save its attributes to the database (Section 7.4). At the end of the signup process, it’s important to render a profile page with the newly created user’s information, so we’ll begin by making a page for showing users, which will serve as the first step toward implementing the REST architecture for users (Section 2.2.2). Along the way, we’ll build on our work in Section 5.3.4 to write succinct and expressive integration tests.
In this chapter, we’ll rely on the User model validations from Chapter 6 to increase the odds of new users having valid email addresses. In Chapter 11, we’ll make sure of email validity by adding a separate account activation step to user signup.
Although this tutorial is designed to be as simple as possible while still being professional-grade, web development is a complicated subject, and Chapter 7 necessarily marks a significant increase in the difficulty of the exposition. I recommend taking your time with the material and reviewing it as necessary. (Some readers have reported simply doing the chapter twice is a helpful exercise.) You might also consider subscribing to the courses at Learn Enough (https://www.learnenough.com/) to gain additional assistance, both with this tutorial and with related Learn Enough titles (especially Learn Enough Ruby to Be Dangerous (https://www.learnenough.com/ruby)).
7.1 Showing Users
In this section, we’ll take the first steps toward the final profile by making a page to display a user’s name and profile photo, as indicated by the mockup in Figure 7.1.1 Our eventual goal for the user profile pages is to show the user’s profile image, basic user data, and a list of microposts, as mocked up in Figure 7.2.2 (Figure 7.2 includes an example of lorem ipsum text, which has a fascinating story that you should definitely read about some time.) We’ll complete this task, and with it the sample application, in Chapter 14.
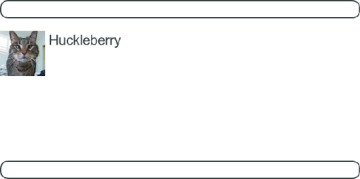
Figure 7.1: A mockup of the user profile made in this section.
If you’re following along with version control, make a topic branch as usual:
$ git checkout -b sign-up
7.1.1 Debug and Rails Environments
The profiles in this section will be the first truly dynamic pages in our application. Although the view will exist as a single page of code, each profile will be customized using information retrieved from the application’s database. As preparation for adding dynamic pages to our sample application, now is a good time to add some debug information to our site layout (Listing 7.1). This displays some useful information about each page using the built-in debug method and params variable (which we’ll learn more about in Section 7.1.2).

Figure 7.2: A mockup of our best guess at the final profile page.
Listing 7.1: Adding some debug information to the site layout.
app/views/layouts/application.html.erb
<!DOCTYPE html> <html> . . . <body> <%= render 'layouts/header' %> <div class="container"> <%= yield %> <%= render 'layouts/footer' %> <%= debug(params) if Rails.env.development? %> </div> </body> </html>
Since we don’t want to display debug information to users of a deployed application, Listing 7.1 uses
if Rails.env.development?
to restrict the debug information to the development environment, which is one of three environments defined by default in Rails (Box 7.1).3 In particular, Rails.env.development? is true only in a development environment, so the embedded Ruby
<%= debug(params) if Rails.env.development? %>
won’t be inserted into production applications or tests. (Inserting the debug information into tests probably wouldn’t do any harm, but it probably wouldn’t do any good, either, so it’s best to restrict the debug display to development only.)
To make the debug output look nicer, we’ll add some rules to the custom stylesheet created in Chapter 5, as shown in Listing 7.2.4
Listing 7.2: Adding code for a prettier debug box.
app/assets/stylesheets/custom.scss
@import "bootstrap-sprockets"; @import "bootstrap"; . . . /* miscellaneous */ .debug_dump { clear: both; float: left; width: 100%; margin-top: 45px; }
The result is shown in Figure 7.3.
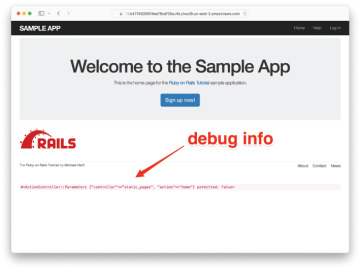
Figure 7.3: The sample application Home page with debug information.
The debug output in Figure 7.3 gives potentially useful information about the page being rendered:
#<ActionController::Parameters {"controller"=>"static_pages", "action"=>"home"} permitted: false>
This is a literal representation of params, which is basically a hash, and in this case identifies the controller and action for the page. We’ll see another example in Section 7.1.2.
The specific representation of the debug information is exactly the kind of thing that might depend on the exact version of Rails, and in fact prior to Rails 7 debug(params) was displayed in the so-called YAML format.5 Because Rails has generally been stable at the level of this tutorial for many years now, some of the screenshots still show this earlier representation (Figure 7.4). Being able to handle such minor discrepancies is a hallmark of technical sophistication (Box 1.2).

Figure 7.4: The debug information in an earlier version of Rails.
Exercises
Visit /about in your browser and use the debug information to determine the controller and action of the params hash.
In the Rails console, pull the first user out of the database and assign it to the variable user. What is the output of puts user.attributes.to_yaml? Compare this to using the y method via y user.attributes.
7.1.2 A Users Resource
In order to make a user profile page, we need to have a user in the database, which introduces a chicken-and-egg problem: How can the site have a user before there is a working signup page? Happily, this problem has already been solved—in Section 6.3.4, we created a User record by hand using the Rails console, so there should be one user in the database:
$ rails console >> User.count => 1 >> User.first => #<User id: 1, name: "Michael Hartl", email: "mhartl@example.com", created_at: "2022-03-11 03:15:38", updated_at: "2022-03-11 03:15:38", password_digest: [FILTERED]>
(If you don’t currently have a user in your database, you should visit Section 6.3.4 now and complete it before proceeding.) We see from the console output above that the user has id 1, and our goal now is to make a page to display this user’s information. We’ll follow the conventions of the REST architecture favored in Rails applications (Box 2.2), which means representing data as resources that can be created, shown, updated, or destroyed—four actions corresponding to the four fundamental operations POST, GET, PATCH, and DELETE defined by the HTTP standard (Box 3.2).
When following REST principles, resources are typically referenced using the resource name and a unique identifier. What this means in the context of users—which we’re now thinking of as a Users resource—is that we should view the user with id 1 by issuing a GET request to the URL /users/1. Here the show action is implicit in the type of request—when Rails’ REST features are activated, GET requests are automatically handled by the show action.
We saw in Section 2.2.1 that the page for a user with id 1 has URL /users/1. Unfortunately, visiting that URL right now just gives an error (Figure 7.5).

Figure 7.5: The current state of /users/1.
We can get the routing for /users/1 to work by adding a single line to our routes file (config/routes.rb):
resources :users
The result appears in Listing 7.3.
Listing 7.3: Adding a Users resource to the routes file.
config/routes.rb
Rails.application.routes.draw do root "static_pages#home" get "/help", to: "static_pages#help" get "/about", to: "static_pages#about" get "/contact", to: "static_pages#contact" get "/signup", to: "users#new" resources :users end
Although our immediate motivation is making a page to show users, the single line resources :users doesn’t just add a working /users/1 URL; it endows our sample application with all the actions needed for a RESTful Users resource,6 along with a large number of named routes (Section 5.3.3) for generating user URLs. The resulting correspondence of URLs, actions, and named routes is shown in Table 7.1. (Compare to Table 2.2.) Over the course of the next three chapters, we’ll cover all of the other entries in Table 7.1 as we fill in all the actions necessary to make Users a fully RESTful resource.
Table 7.1: RESTful routes provided by the Users resource in Listing 7.3.
HTTP request method |
URL |
Action |
Named route |
Purpose |
---|---|---|---|---|
GET |
/users |
index |
users_path |
page to list all users |
GET |
/users/1 |
show |
user_path(user) |
page to show user |
GET |
/users/new |
new |
new_user_path |
page to make a new user (signup) |
POST |
/users |
create |
users_path |
create a new user |
GET |
/users/1/edit |
edit |
edit_user_path(user) |
page to edit user with id 1 |
PATCH |
/users/1 |
update |
user_path(user) |
update user |
DELETE |
/users/1 |
destroy |
user_path(user) |
delete user |
With the code in Listing 7.3, the routing works, but there’s still no page there (Figure 7.6). To fix this, we’ll begin with a minimalist version of the profile page, which we’ll flesh out in Section 7.1.4.
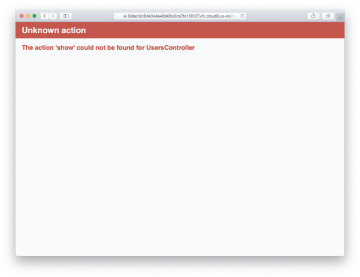
Figure 7.6: The URL /users/1 with routing but no page.
We’ll use the standard Rails location for showing a user, which is app/views/users/show.html.erb. Unlike the new.html.erb view, which we created with the generator in Listing 5.38, the show.html.erb file doesn’t currently exist, so you’ll have to create it by hand,7 and then fill it with the content shown in Listing 7.4.
Listing 7.4: A stub view for showing user information.
app/views/users/show.html.erb
<%= @user.name %>, <%= @user.email %>
This view uses embedded Ruby to display the user’s name and email address, assuming the existence of an instance variable called @user. Of course, eventually the real user show page will look very different (and won’t display the email address publicly).
In order to get the user show view to work, we need to define an @user variable in the corresponding show action in the Users controller. As you might expect, we use the find method on the User model (Section 6.1.4) to retrieve the user from the database, as shown in Listing 7.5.
Listing 7.5: The Users controller with a show action.
app/controllers/users_controller.rb
class UsersController < ApplicationController def show @user = User.find(params[:id]) end def new end end
Here we’ve used params to retrieve the user id. When we make the appropriate request to the Users controller, params[:id] will be the user id 1, so the effect is the same as the find method User.find(1) we saw in Section 6.1.4. (Technically, params[:id] is the string "1", but find is smart enough to convert this to an integer.)
With the user view and action defined, the URL /users/1 works perfectly, as seen in Figure 7.7. (If you haven’t restarted the Rails server since adding bcrypt, you may have to do so at this time. This sort of thing is a good application of technical sophistication (Box 1.2).) Note that the debug information in Figure 7.7 confirms the value of params[:id]:
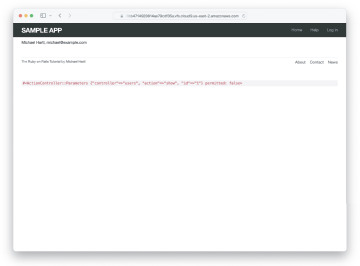
Figure 7.7: The user show page after adding a Users resource.
{"controller"=>"users", "action"=>"show", "id"=>"1"}
This is why the code
User.find(params[:id])
in Listing 7.5 finds the user with id 1.
Exercises
Using embedded Ruby, add the created_at and updated_at “magic column” attributes to the user show page from Listing 7.4.
Using embedded Ruby, add Time.now to the user show page. What happens when you refresh the browser?
7.1.3 Debugger
We saw in Section 7.1.2 how the information from the debug method could help us understand what’s going on in our application, but there’s also a more direct way to get debugging information. To see how it works, we just need to add a line consisting of debugger to our application, as shown in Listing 7.6.
Listing 7.6: The Users controller with a debugger.
app/controllers/users_controller.rb
class UsersController < ApplicationController def show @user = User.find(params[:id]) debugger end def new end end
Now, when we visit /users/1, the Rails server shows an rdbg (Ruby debugger) prompt (Figure 7.8):

Figure 7.8: The debugger prompt in the Rails server.
(rdbg)
We can treat the debugger like a Rails console, issuing commands to figure out the state of the application:
(rdbg) @user.name "Michael Hartl" (rdbg) @user.email "michael@example.com" (rdbg) params[:id] "1"
To release the prompt and continue execution of the application, press Ctrl-D, then remove the debugger line from the show action (Listing 7.7).
Listing 7.7: The Users controller with the debugger line removed.
app/controllers/users_controller.rb
class UsersController < ApplicationController def show @user = User.find(params[:id]) end def new end end
Whenever you’re confused about something in a Rails application, it’s a good practice to put debugger close to the code you think might be causing the trouble. Inspecting the state of the system using byebug is a powerful method for tracking down application errors and interactively debugging your application.
Exercises
With the debugger in the show action as in Listing 7.6, hit /users/1. Use puts to display the value of the YAML form of the params hash. Hint: Refer to the relevant exercise in Section 7.1.1. How does it compare to the debug information shown by the debug method in the site template?
Put the debugger in the User new action and hit /users/new. What is the value of @user?
7.1.4 A Gravatar Image and a Sidebar
Having defined a basic user page in the previous section, we’ll now flesh it out a little with a profile image for each user and the first cut of the user sidebar. We’ll start by adding a “globally recognized avatar”, or Gravatar, to the user profile.8 Gravatar is a free service that allows users to upload images and associate them with email addresses they control. As a result, Gravatars are a convenient way to include user profile images without going through the trouble of managing image upload, cropping, and storage; all we need to do is construct the proper Gravatar image URL using the user’s email address and the corresponding Gravatar image will automatically appear. (We’ll learn how to handle custom image upload in Section 13.4.)
Our plan is to define a gravatar_for helper function to return a Gravatar image for a given user, as shown in Listing 7.8.
Listing 7.8: The user show view with name and Gravatar.
app/views/users/show.html.erb
<% provide(:title, @user.name) %> <h1> <%= gravatar_for @user %> <%= @user.name %> </h1>
By default, methods defined in any helper file are automatically available in any view, but for convenience we’ll put the gravatar_for method in the file for helpers associated with the Users controller. As noted in the Gravatar documentation, Gravatar URLs are based on an MD5 hash of the user’s email address. In Ruby, the MD5 hashing algorithm is implemented using the hexdigest method, which is part of the Digest library:
>> email = "MHARTL@example.COM" >> Digest::MD5::hexdigest(email.downcase) => "1fda4469bcbec3badf5418269ffc5968"
Since email addresses are case-insensitive (Section 6.2.4) but MD5 hashes are not, we’ve used the downcase method to ensure that the argument to hexdigest is all lowercase. (Because of the email downcasing callback in Listing 6.32, this will never make a difference in this tutorial, but it’s a good practice in case the gravatar_for method ever gets used on email addresses from other sources.) The resulting gravatar_for helper appears in Listing 7.9.
Listing 7.9: Defining a gravatar_for helper method.
app/helpers/users_helper.rb
module UsersHelper # Returns the Gravatar for the given user. def gravatar_for(user) gravatar_id = Digest::MD5::hexdigest(user.email.downcase) gravatar_url = "https://secure.gravatar.com/avatar/#{gravatar_id}" image_tag(gravatar_url, alt: user.name, class: "gravatar") end end
The code in Listing 7.9 returns an image tag for the Gravatar with a gravatar CSS class and alt text equal to the user’s name (which is especially convenient for visually impaired users using a screen reader).
The resulting profile page should appear as in Figure 7.9. The displayed Gravatar image is a generic default because michael@example.com isn’t a real email address and hence can’t be associated with a custom Gravatar. (In fact, as you can see by visiting it, the example.com domain is reserved for examples just like this one.) By the way, if you completed the exercises in Section 5.1.2, be sure to remove the CSS from Listing 5.11 so that the gravatar image displays correctly.
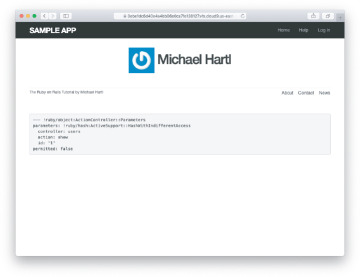
Figure 7.9: The user profile page with the default Gravatar.
To get our application to display a custom Gravatar, we’ll use the update method (Section 6.1.5) to change the user’s email to something I control:9
$ rails console >> user = User.first >> user.update(name: "Example User", ?> email: "example@railstutorial.org", ?> password: "foobar", ?> password_confirmation: "foobar") => true
Here we’ve assigned the user the email address example@railstutorial.org, which I’ve associated with the Rails Tutorial logo, as seen in Figure 7.10.
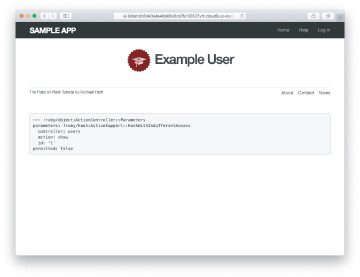
Figure 7.10: The user show page with a custom Gravatar.
The last element needed to complete the mockup from Figure 7.1 is the initial version of the user sidebar. We’ll implement it using the aside tag, which is used for content (such as sidebars) that complements the rest of the page but can also stand alone. We include row and col-md-4 classes, which are both part of Bootstrap. The code for the modified user show page appears in Listing 7.10.
Listing 7.10: Adding a sidebar to the user show view.
app/views/users/show.html.erb
<% provide(:title, @user.name) %> <div class="row"> <aside class="col-md-4"> <section class="user_info"> <h1> <%= gravatar_for @user %> <%= @user.name %> </h1> </section> </aside> </div>
With the HTML elements and CSS classes in place, we can style the profile page (including the sidebar and the Gravatar) with the SCSS shown in Listing 7.11.10 (Note the nesting of the table CSS rules, which works only because of the Sass engine used by the asset pipeline.) The resulting page is shown in Figure 7.11.

Figure 7.11: The user show page with a sidebar and CSS.
Listing 7.11: SCSS for styling the user show page, including the sidebar.
app/assets/stylesheets/custom.scss
. . . /* sidebar */ aside { section.user_info { margin-top: 20px; } section { padding: 10px 0; margin-top: 20px; &:first-child { border: 0; padding-top: 0; } span { display: block; margin-bottom: 3px; line-height: 1; } h1 { font-size: 1.4em; text-align: left; letter-spacing: -1px; margin-bottom: 3px; margin-top: 0px; } } } .gravatar { float: left; margin-right: 10px; } .gravatar_edit { margin-top: 15px; }
Exercises
Associate a Gravatar with your primary email address if you haven’t already. What is the MD5 hash associated with the image?
Verify that the code in Listing 7.12 allows the gravatar_for helper defined in Section 7.1.4 to take an optional size parameter, allowing code like gravatar_for user, size: 50 in the view. (We’ll put this improved helper to use in Section 10.3.1.)
The options hash used in the previous exercise is still commonly used, but as of Ruby 2.0 we can use keyword arguments instead. Confirm that the code in Listing 7.13 can be used in place of Listing 7.12. What are the diffs between the two?
Listing 7.12: Adding an options hash in the gravatar_for helper.
app/helpers/users_helper.rb
module UsersHelper # Returns the Gravatar for the given user. def gravatar_for(user, options = { size: 80 }) size = options[:size] gravatar_id = Digest::MD5::hexdigest(user.email.downcase) gravatar_url = "https://secure.gravatar.com/avatar/#{gravatar_id}?s=#{size}" image_tag(gravatar_url, alt: user.name, class: "gravatar") end end
Listing 7.13: Using keyword arguments in the gravatar_for helper.
app/helpers/users_helper.rb
module UsersHelper # Returns the Gravatar for the given user. def gravatar_for(user, size: 80) gravatar_id = Digest::MD5::hexdigest(user.email.downcase) gravatar_url = "https://secure.gravatar.com/avatar/#{gravatar_id}?s=#{size}" image_tag(gravatar_url, alt: user.name, class: "gravatar") end end