Create a GUI with Java
- Creating an Application
- Working with Components
- Lists
- The Java Class Library
- Summary
- Q and A
- Quiz
- Certification Practice
- Exercises
Walks you through how to use Swing to create applications that feature key GUI components.
Most computer users today expect software to feature a graphical user interface (GUI) with a variety of widgets such as text boxes, sliders, and scrollbars. The Java Class Library includes Swing, a set of packages that enable Java programs to offer a sophisticated GUI and collect user input with the mouse, keyboard, and other input devices.
In this lesson, you will use Swing to create applications that feature these GUI components:
Frames: Windows with a title bar; menu bar; and Maximize, Minimize, and Close buttons
Containers: Components that hold other components
Buttons: Clickable rectangles with text or graphics indicating their purpose
Labels: Text or graphics that provide information
Text fields and text areas: Windows that accept keyboard input of one line or multiple lines
Drop-down lists: Groups of related items that are selected from drop-down menus or scrolling windows
Check boxes and radio buttons: Small squares or circles that can be selected or deselected
Image icons: Graphics added to buttons, labels, and other components
Scrolling panes: Panels for components too big for a user interface that can be accessed in full by using a scrollbar
Swing is the most extensive set of related classes introduced thus far in the book. Learning to create graphical applications with these packages is good practice for utilizing a class library in Java, which is something you’ll do often in your own projects.
Creating an Application
Swing enables the creation of a Java program with an interface that adopts the style of the native operating system, such as Windows or Linux, or a style that’s unique to Java. Each of these styles is called a look and feel because it describes both the appearance of the interface and how its components function when they are used.
Java offers a distinctive look and feel called Nimbus that’s unique to the language.
Swing components are part of the javax.swing package, a standard part of the Java Class Library. To refer to a Swing class using its short name—without referring to the package, in other words—you must make it available with an import statement or use a catchall statement such as the following:
import javax.swing.*;
Two other packages that support GUI programming are java.awt, the Abstract Window Toolkit (AWT), and java.awt.event, event-handling classes that handle user input.
When you use a Swing component, you work with objects of that component’s class. You create the component by calling its constructor and then calling methods of the component as needed for proper setup.
All Swing components are subclasses of the abstract class JComponent. It includes methods to set a component’s size, change the background color, define the font used for any displayed text, and set up tooltips. A tooltip is explanatory text that appears when you hover the mouse over the component for a few seconds.
Before components can be displayed in a user interface, they must be added to a container, a component that can hold other components. Swing containers are subclasses of java.awt.Container. This class includes methods to add and remove components from a container, arrange components using an object called a layout manager, and set up borders around the edges of a container. Containers often can be placed in other containers.
Creating a Graphical User Interface
The first step in creating a Swing application is to create a class that represents the main GUI. An object of this class serves as a container that holds all the other components to be displayed.
In many projects, the main interface object is a frame (the JFrame class in the javax.swing package). A frame is a window shown whenever you open an application on your computer. A frame has a title bar; Maximize, Minimize, and Close buttons; and other features.
In a graphical environment such as Windows or macOS, users expect to be able to move, resize, and close the windows of programs. One way to create a graphical Java application is to make the interface a subclass of JFrame, as in the following class declaration:
public class FeedReader extends JFrame { // body of class }
The constructor of the class should handle the following tasks:
Call a superclass constructor with super() to give the frame a title and handle other setup procedures.
Set the size of the frame’s window, either by specifying the width and height in pixels or by letting Swing choose the size.
Decide what to do if a user closes the window.
Display the frame.
The JFrame class has the simple constructors JFrame() and JFrame(String). One sets the frame’s title bar to the specified text, and the other leaves the title bar empty. You also can set the title by calling the frame’s setTitle(String) method.
The size of a frame can be established by calling the setSize(int, int) method with the width and height as arguments. A frame’s size is indicated in pixels, so, for example, calling setSize(650, 550) creates a frame 650 pixels wide and 550 pixels tall.
Another way to set a frame’s size is to fill the frame with the components it will contain and then call the frame’s pack() method. This resizes the frame based on the preferred size of the components inside it. If the frame is bigger than it needs to be, pack() shrinks it to the minimum size required to display the components. If the frame is too small (or the size has not been set), pack() expands it to the required size.
Frames are invisible when they are created. You can make them visible by calling the frame’s setVisible(boolean) method with the literal true as an argument.
If you want a frame to be displayed when it is created, call one of these methods in the constructor. You also can leave the frame invisible and require any class that uses the frame to make it visible by calling setVisible(true). As you probably have surmised, calling setVisible(false) makes a frame invisible.
When a frame is displayed, the default behavior is for it to be positioned in the upper-left corner of the computer’s desktop. You can specify a different location by calling the setBounds(int, int, int, int) method. The first two arguments to this method are the (x, y) position of the frame’s upper-left corner on the desktop. The last two arguments are the frame’s width and height.
Another way to set the bounds is with a Rectangle object from the java.awt package. Create the rectangle with the Rectangle(int, int, int, int) constructor. The first two arguments are the (x, y) position of the upper-left corner. The next two are the width and height. Call setBounds(Rectangle) to draw the frame at that spot.
The following class represents a 300×100 frame with “Edit Payroll” in the title bar:
public class Payroll extends JFrame { public Payroll() { super("Edit Payroll"); setSize(300, 100); setVisible(true); } }
Every frame has Maximize, Minimize, and Close buttons on the title bar that the user can control—the same controls present in the interface of other software running on your computer.
There’s a wrinkle to using frames that you might not expect: The normal behavior when a frame is closed is for the application to keep running. When a frame serves as a program’s main GUI, this leaves a user with no way to stop the program.
To change this, you must call a frame’s setDefaultCloseOperation(int) method with one of four static variables as an argument:
EXIT_ON_CLOSE: Exits the application when the frame is closed
DISPOSE_ON_CLOSE: Closes the frame, removes the frame object from Java Virtual Machine (JVM) memory, and keeps running the application
DO_NOTHING_ON_CLOSE: Keeps the frame open and continues running
HIDE_ON_CLOSE: Closes the frame and continues running
These variables are part of the JFrame class because it implements the WindowConstants interface. To prevent a user from closing a frame, add the following statement to the frame’s constructor method:
setDefaultCloseOperation(JFrame.DO_NOTHING_ON_CLOSE);
If you are creating a frame to serve as an application’s main user interface, the expected behavior is probably EXIT_ON_CLOSE, which shuts down the application along with the frame.
As mentioned earlier, you can customize the overall appearance of a user interface in Java by designating a look and feel. The UIManager class in the javax.swing package manages this aspect of Swing. To set the look and feel, call the class method setLookAndFeel(String) with the name of the look and feel’s class as the argument. Here’s how to choose the Nimbus look and feel:
UIManager.setLookAndFeel( "javax.swing.plaf.nimbus.NimbusLookAndFeel" );
This method call should be contained within a try-catch block because it might generate five different exceptions. Catching the Exception class and ignoring it causes the default look and feel to be used in the unlikely circumstance that Nimbus can’t be chosen properly.
Developing a Framework
This lesson’s first project is an application that displays a frame containing no other interface components. In NetBeans, create a new Java file with the class name SimpleFrame and the package name com.java21days and then enter Listing 9.1 as the source code. This simple application displays a frame 300×100 pixels in size and can serve as a framework—pun unavoidable—for any applications you create that use a GUI.
LISTING 9.1 The Full Text of SimpleFrame.java
1: package com.java21days; 2: 3: import javax.swing.*; 4: 5: public class SimpleFrame extends JFrame { 6: public SimpleFrame() { 7: super("Frame Title"); 8: setSize(300, 100); 9: setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); 10: setVisible(true); 11: } 12: 13: private static void setLookAndFeel() { 14: try { 15: UIManager.setLookAndFeel( 16: "javax.swing.plaf.nimbus.NimbusLookAndFeel" 17: ); 18: } catch (Exception exc) { 19: // ignore error 20: } 21: } 22: 23: public static void main(String[] arguments) { 24: setLookAndFeel(); 25: SimpleFrame sf = new SimpleFrame(); 26: } 27: }
When you compile and run the application, you should see the frame displayed in Figure 9.1.

FIGURE 9.1 Displaying a frame.
The SimpleFrame application isn’t much to look at. The GUI contains no components, aside from the standard Minimize, Maximize, and Close (X) buttons on the title bar, as shown in Figure 9.1. You’ll add components later in this lesson.
In the application, a SimpleFrame object is created in the main() method in lines 23–26. If you had not displayed the frame when it was constructed, you could call sf.setVisible(true) in the main() method to display the frame.
Nimbus is set as the frame’s look and feel in lines 15–17.
The work involved in creating the frame’s user interface takes place in the SimpleFrame() constructor in lines 6–11. Components can be created and added to the frame within this constructor.
Creating a Component
Creating a GUI is a great way to get experience working with objects in Java because each interface component is represented by its own class.
To use an interface component in Java, you create an object of that component’s class. You already have worked with the container class JFrame.
One of the simplest components to employ is JButton, the class that represents clickable buttons.
In any program, buttons trigger actions. You could click Install to begin installing software, click a Run button to begin a new game of Angry Birds, click the Minimize button to prevent your boss from seeing Angry Birds running, and so on.
A Swing button can feature a text label, a graphical icon, or both.
You can use the following constructors for buttons:
JButton(String): A button labeled with the specified text
JButton(Icon): A button that displays the specified graphical icon
JButton(String, Icon): A button with the specified text and graphical icon
The following statements create three buttons with text labels:
JButton play = new JButton("Play"); JButton stop = new JButton("Stop"); JButton rewind = new JButton("Rewind");
Graphical buttons with icons are covered later in this lesson.
Adding Components to a Container
Before you can display a user interface component such as a button in a Java program, you must add it to a container and display that container.
To add a component to a container, call the container’s add(Component) method with the component as the argument. (All user interface components in Swing inherit from java.awt.Component.)
The simplest Swing container is a panel (the JPanel class). The following example creates a button and adds it to a panel:
JButton quit = new JButton("Quit"); JPanel panel = new JPanel(); panel.add(quit);
Use the same technique to add components to frames and windows.
The ButtonFrame class, shown in Listing 9.2, expands on the application framework created earlier in this lesson. A panel is created, three buttons are added to the panel, and then the panel is added to a frame. Enter the source code of Listing 9.2 into a new Java file called ButtonFrame in NetBeans, making sure to put it in the com.java21days package.
LISTING 9.2 The Full Text of ButtonFrame.java
1: package com.java21days; 2: 3: import javax.swing.*; 4: 5: public class ButtonFrame extends JFrame { 6: JButton load = new JButton("Load"); 7: JButton save = new JButton("Save"); 8: JButton unsubscribe = new JButton("Unsubscribe"); 9: 10: public ButtonFrame() { 11: super("Button Frame"); 12: setSize(340, 170); 13: setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); 14: JPanel pane = new JPanel(); 15: pane.add(load); 16: pane.add(save); 17: pane.add(unsubscribe); 18: add(pane); 19: setVisible(true); 20: } 21: 22: private static void setLookAndFeel() { 23: try { 24: UIManager.setLookAndFeel( 25: "javax.swing.plaf.nimbus.NimbusLookAndFeel" 26: ); 27: } catch (Exception exc) { 28: System.out.println(exc.getMessage()); 29: } 30: } 31: 32: public static void main(String[] arguments) { 33: setLookAndFeel(); 34: ButtonFrame bf = new ButtonFrame(); 35: } 36: }
When you run the application, a small frame opens that contains the three buttons, as shown in Figure 9.2.
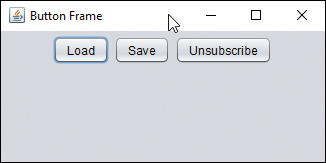
FIGURE 9.2 The ButtonFrame application.
The ButtonFrame class has three instance variables: the load, save, and unsubscribe JButton objects.
In lines 14–17 of Listing 9.2, a new JPanel object is created, and the three buttons are added to the panel by calls to its add(Component) method. When the panel contains all the buttons, the frame’s own add(Component) method is called in line 18 with the panel as an argument, and the panel is added to the frame.