- Choose a Text Editor
- Making Your Choice
- Create a Project Directory
- Start Small: Create a Test File
- Build on Your Success
- Great Power, Great Responsibility
- Summing Up
Start Small: Create a Test File
Now that you have your project directory, let’s start programming. The first thing you’re going to do is create an HTML file in your kittenbook directory called kittenbook.html. You can create a new file in a few different ways, but the easiest is to open your chosen text editor, create a new file (click File → New File), and then save it as kittenbook.html (File → Save As, then find your kittenbook directory, type in kittenbook.html as the filename, and click Save). Now fill in kittenbook.html with the code in Listing 1.1.
Listing 1.1 kittenbook.html
<html> <head> <title>My First Program</title> </head> <body> <p>Hello, World!</p> </body> </html>
Save the file and then open it in a web browser by double-clicking it in your file browser. You should see something similar to Figure 1.4.
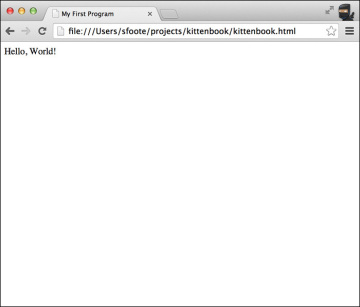
Figure 1.4 You told the computer to do something, and it worked!
Take a break to enjoy this moment. Now look back at your code and compare it to what you see in the web browser. You should see Hello, World! in the window body, and you should see My First Program in the tab. HTML (Hypertext Markup Language) is made up of elements. An element consists of an opening tag, an optional body, and a closing tag. For instance <p> is an opening tag for the paragraph element, Hello, World is the content, and </p> is the closing tag. The body of an element can also contain other elements. For instance, see Listing 1.2.
Listing 1.2 The <head> Tag of kittenbook.html
<head> <title>My First Program</title> </head>
The head element contains a title element. This tells the web browser the title of the HTML page, and most web browsers display that title in the tab. You have plenty more to learn about HTML, but we’ll leave it at that for now.
How HTML and JavaScript Work Together in a Browser
You’ve just created your first web page. Now let’s make the page a little more interactive. Create another file in your kittenbook directory called kittenbook.js. This will be a JavaScript file, and it will make your web page more interesting.
Open kittenbook.js with your text editor and fill it with the code in Listing 1.3.
Listing 1.3 Hello, Friend!
alert('Hello, [your name]!');
Replace [your name] with your real name. For me, this would be alert('Hello, Steven!').
Now open your web page again (or reload it) and see what happens. It’s a trick! The page should look exactly the same as it did before. The HTML doesn’t know that the JavaScript exists, and that’s because we didn’t tell the HTML about the JavaScript. Update kittenbook.html by adding the line from Listing 1.4 inside the <body> element.
Listing 1.4 Add Your JavaScript to kittenbook.html
<script type="text/javascript" src="kittenbook.js"></script>
Now kittenbook.html should look like Listing 1.5.
Listing 1.5 kittenbook.html with JavaScript
<html> <head> <title>My First Program</title> </head> <body> <p>Hello, World!</p> <script type="text/javascript" src="kittenbook.js"></script> </body> </html>
This time, you should see something similar to Figure 1.5.
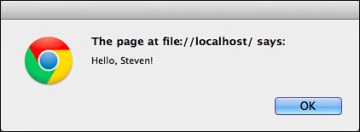
Figure 1.5 Hello, you wrote a real program!
That was awesome! You made a window appear with your name in it. You could type whatever you wanted, and it would show up in that window. Let’s talk about what you just did; then we’ll take it to the next level.
The JavaScript you wrote is a single instruction for your computer. alert is something called a function that tells the web browser to open a window with an OK button. You can add some text to the window by adding an argument to alert. A function is a piece of code that can be called to perform a task. An argument can modify how the function performs the task. The semicolon at the end of the line indicates to JavaScript the end of an instruction. Programs usually have many instructions, so the semicolons separate the instructions.
The Value of Small Changes
Now we’re going to enhance our program so that we can say hello to anyone, instead of just “Hello, World!” As you make the enhancements, make small changes. Then test those small changes before you make more changes. At first, this process might seem tedious and unnecessary. However, if you make many changes before testing and find that your program isn’t working, you will have a hard time determining which of your changes broke the program. If you make one small change before testing and see that your program is broken, you will know exactly what you did to break it.
Our first small change is to ask for a name. A personalized greeting isn’t much good without a name. For this example, we use a function called prompt because it is simple and it works well here. However, I beg you not to use prompt (or alert) with a real website. Although prompt and alert are convenient for testing and learning, they make for a poor and outdated user experience. We cover alternatives for them in Chapter 8, “Functions and Methods.” For now, update your kittenbook.js to use prompt instead of alert, and change the message to say “Hello, what’s your name?” (see Listing 1.6).
Listing 1.6 Prompt for a Name
prompt('Hello, what\'s your name?');
You might be wondering why this code says what\'s instead of what's. A group of characters such as 'Hello, World!' is called a string. A string starts with a quote and ends with a quote. If I type prompt('Hello, what's your name?');, then I have three quotes (even though one of them is intended to be an apostrophe), which confuses the computer. The computer sees the string 'Hello, what', then sees some other characters that it doesn’t understand, and then sees another string: ');'. I use a backslash before the single quote in what\'s to tell the computer that I don’t mean the apostrophe to signify the end of the string. The backslash is called an escape character.
Now if you refresh your page, you should see a window with a place for you to type your name (see Figure 1.6).

Figure 1.6 What’s your name?
Notice that when you type in your name and click OK, the page still says Hello, World! What gives? Why ask for a name if you’re not going to show it? Well, we haven’t told the computer what to do with the name. We made a small change: We switched the alert window for a prompt window. It worked, so now we can tell the computer what to do with the name.
One of the most important tools in programming is the variable. A variable is a place to store some data that affects how the program works—Chapter 5, “Data (Types), Data (Structures), Data(bases),” discusses variables in great detail. In this case, we want to store the name from the prompt window. (See Listing 1.7.)
Listing 1.7 Your First Variable
var userName = prompt('Hello, what\'s your name?');
All variables have names, and we have called our variable userName. To tell the computer that we are creating a variable, we use a reserved word: var. A reserved word is a word that has special meaning to a programming language. It is reserved, so you cannot use that word as the name of a variable; you don’t want to confuse your computer.
Now that we have stored our variable, refresh the page to see that everything still works. The web page should look the same as the last time you refreshed. We have stored the username, but we haven’t told the computer to do anything with it yet. The next step is to insert the username in the web page (see Listing 1.8).
Listing 1.8 Put Your Variable to Work
var userName = prompt('Hello, what\'s your name?');
document.body.innerHTML = 'Hello, ' + userName + '!';
We add a new instruction to change the HTML document’s body. Remember the <body> element from kittenbook.html? document.body.innerHTML refers to everything between the opening tag and the closing tag of the <body> element. document.body.innerHTML = 'Hello, ' + userName + '!'; effectively changes the HTML to look like Listing 1.9 (assuming that I type Steven when the prompt asks for my name).
Listing 1.9 kittenbook.html When Your JavaScript Is Done with It
<html> <head> <title>My First Program</title> </head> <body> Hello, Steven! </body> </html>
Congratulations! You just created a real program. Admittedly, this is not the most useful program that has ever been written, but it is certainly not the least useful—and you learned a lot along the way.