Creating a Windows 8 App: Try Out File | New | Project
When learning a new technology, going to File | New | Project can be beneficial, to see what the options are. Sometimes we have many options; other times, we have only one. After selecting a project, it is fun to start digging in and seeing what kind of havoc can be wrought.
When we run Visual Studio 2012 and select File | New | Project, we can see the Windows Store option under the JavaScript section of the templates. From here, we have five different project templates to choose from (see Figure 2.1). In this hour, we dive into all these templates. We also discuss page navigation in Windows Store apps and discuss how to load content from outside an app. We look at the different security context our apps run in. Finally, we examine how to pass data back and forth to the different contexts.
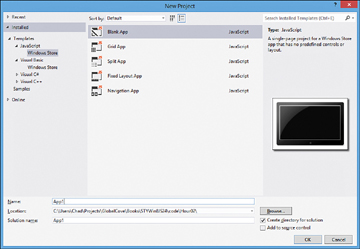
Figure 2.1. Visual Studio offers five JavaScript Windows Store app project template choices.
Creating a Blank App Project
The first project template we create is the Blank App. In creating this app, we can see that it isn’t really blank. Figure 2.2 shows the Solution Explorer immediately after creating the project.

Figure 2.2. The Blank App project isn’t so blank after all.
Let’s take a moment to look at these files and explain what each one does. To begin, we open default.html. As expected, this is the main page that will run. The start page is determined from package.appxmanifest. We discuss this file shortly in the section on app manifest.
Examining the Blank App HTML File
Inside the default.html file is the following markup:
<!
DOCTYPE
html
>
<
html
>
<
head
>
<
meta
charset
="utf-8"
/>
<
title
>
BlankApp
</
title
>
<!-- WinJS references -->
<
link
href
="//Microsoft.WinJS.1.0/css/ui-dark.css"
rel
="stylesheet"
/>
<
script
src
="//Microsoft.WinJS.1.0/js/base.js"></
script
>
<
script
src
="//Microsoft.WinJS.1.0/js/ui.js"></
script
>
<!-- BlankApp references -->
<
link
href
="/css/default.css"
rel
="stylesheet"
/>
<
script
src
="/js/default.js"></
script
>
</
head
>
<
body
>
<
p
>
Content goes here
</
p
>
</
body
>
</
html
>
The project was named BlankApp, so Visual Studio used that as the page title. Everything else is the same whenever a new Blank App project is created. Everything is as expected: The HTML5 doctype is followed by the html and head and body elements. Inside the head element are references to some CSS files and JavaScript files. Under the comment <!-- WinJS references --> is the ui-dark.css reference.
Referencing the Windows Library for JavaScript
Following the CSS reference are two scripts, base.js and ui.js. The CSS file and the JavaScript files are all part of the Windows Library for JavaScript (WinJS). These files are read-only and should not be modified. The base.js file must be loaded before the ui.js file. If any code is relying on WinJS, both of those files must be loaded before the app’s JavaScript files are. We talk about WinJS in detail during Hour 3, “Exploring the Windows Runtime (WinRT) and Windows Library for JavaScript (WinJS).”
Examining the Blank App CSS File
After referencing the WinJS files, default.html references to the app’s own CSS and JavaScript files. The contents of the Blank App’s default.css is as follows:
body
{
}
@media
screen
and
(
-ms-view-state
:
fullscreen
-landscape)
{
}
@media
screen
and
(
-ms-view-state
:
filled)
{
}
@media
screen
and
(
-ms-view-state
:
snapped)
{
}
@media
screen
and
(
-ms-view-state
:
fullscreen
-portrait)
{
}
Some styles have been stubbed out, but nothing is actually styled. The CSS3 Media Queries (@media) are discussed in detail during Hour 13, “Working with Fullscreen, Filled, Snapped, and Portrait Views.” For now, it is important to know that we can override styles in those sections, to have the app lay out content differently based on portrait view or snapped view.
Examining the Blank App JavaScript File
Listing 2.1 shows the default.js code included in the app. Note that the code is wrapped in a self-executing anonymous function. If the concept of a self-executing anonymous function is unfamiliar, read Hour 1 now. (“GO TO →” Hour 1, Getting a Refresher on JavaScript, HTML5, and CSS3; Self-Executing Anonymous Functions”)
Listing 2.1. default.js from the Blank App
(
function
() {
"use strict"
;
WinJS.
Binding.
optimizeBindingReferences=
true
;
var
app=
WinJS.
Application;
var
activation=
Windows.
ApplicationModel.
Activation;
app.
onactivated=
function
(
args) {
if
(
args.
detail.
kind===
activation.
ActivationKind.
launch) {
if
(
args.
detail.
previousExecutionState!==
activation.
ApplicationExecutionState.
terminated) {
// TODO: This application has been newly launched. Initialize
// your application here.
}
else
{
// TODO: This application has been reactivated from suspension.
// Restore application state here.
}
args.
setPromise(
WinJS.
UI.
processAll());
}
};
app.
oncheckpoint=
function
(
args) {
// TODO: This application is about to be suspended. Save any state
// that needs to persist across suspensions here. You might use the
// WinJS.Application.sessionState object, which is automatically
// saved and restored across suspension. If you need to complete an
// asynchronous operation before your application is suspended, call
// args.setPromise().
};
app.
start();
})();
We dig into this JavaScript in detail during Hour 3. For now, we can see the places in code we can tie into when the app is starting up. We will discuss the application life cycle during Hour 15, “Handling Our App Life Cycle Events”. So far, we have looked at the HTML, the CSS, and the JavaScript. Before we go any further, let’s take a look at the app manifest file.
Examining the Blank App Manifest File
When we open the app manifest file (package.appxmanifest), Visual Studio has a tabbed form where we can enter information. This can be seen in Figure 2.3. Again, we see that the start page is set to default.html. If we wanted the start page to be something different, we would change that setting here. We discuss this XML file in detail during Hour 9, “Working with Location and Hardware Sensors.” For now, let’s look at the different fields available for setting images. On the Application UI tab, a logo file, a small logo file, and a splash screen image file can all be set. These were all provided under the /images folder. In addition, the store logo can also be set under the Packaging tab. We discuss this tab during Hour 23, “Getting Through Certification.”

Figure 2.3. The Application UI tab in the app manifest enables us to set the logos and splash screen images.
Certificates and Keys
The only file left to discuss is the BlankApp_TemporaryKey.pfx file. Windows Store apps must have their manifests digitally signed by a certificate to run. This TemporaryKey file is a Personal Information Exchange file that allows for release signing. The certificate is used for development purposes. If the file gets deleted or another issue arises, the certificate can be generated again by selecting Choose Certificate and then, in the Configure Certificate drop-down, selecting Create Test Certificate (see Figure 2.4).
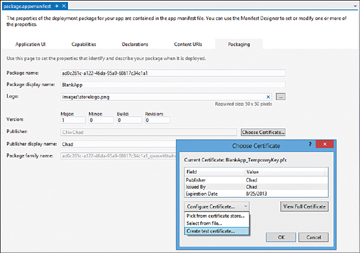
Figure 2.4. The Packaging tab in the app manifest enables us to generate a new test certificate.
Finally, as in Figure 2.5, enter a password and confirmation to generate a new test certificate.
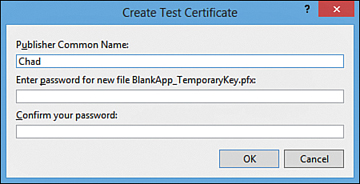
Figure 2.5. A password is needed to finish creating the test certificate.
Figure 2.6 shows the result of running the app.

Figure 2.6. The Blank App produces these exciting results.