Building a Game from Scratch, Part 2: Coding the Game Logic
Hello again. This is the second of a two-part article series in which we use the MIT Scratch development environment to build a video game from…well, scratch.
If you haven’t read the first article in the series, then I strongly suggest that you do so before studying this piece; I want to ensure that you have the proper context for what we’re doing.
The first iteration of our case study project, “Space Game,” can be found at the Scratch website.
The game at this point involves the following play mechanics:
- Click the Green Flag to start the game.
- Use the keyboard arrow keys to fly the Spaceship around the playfield.
- Press SPACE to fire the cannon.
By the end of this article, we’ll add a bunch of additional functionality to the game. Specifically, we’ll:
- Add “enemy” sprites to the playfield.
- Program the game such that it ends if your Spaceship makes contact with an enemy sprite.
- Allow the Spaceship to destroy enemy sprites with its laser cannon.
If you want to get a sneak peek at the second iteration of Space Game, I have one published to the online Scratch Project Gallery.
An in-game screenshot from Space Game 2 is shown in Figure 1.
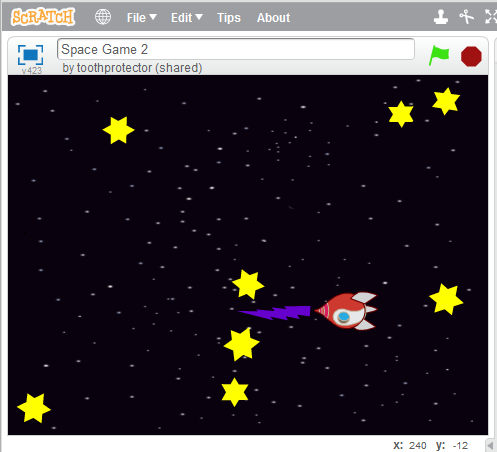
Figure 1 The Space Game 2 in action
In the meantime, we have a lot of work to do—let’s begin!
Adding the Star Sprite
Because our Space Game is intended to pay tribute to classic space shooter games like Atari Asteroids, we need to add an “enemy” sprite that will serve as a target for our Spaceship’s laser cannon.
Open up your active Scratch project in the online editor (I gave you instructions for doing this, as well as a nutshell tour of the editor interface, in Part 1 of this series; you can also purchase my Sams Teach Yourself Scratch 2.0 in 24 Hours book if you’d like end-to-end instruction).
Open the Sprite Library and add an instance of the Star2 shape from the Things category to your project. Rename the sprite Star. So far, so good.
We have three main challenges to overcome in coding the game logic behind the Star sprite:
- We need to spawn multiple copies of the Star to fill the playfield and give the player a challenge.
- We need the stars to appear on-screen in random locations.
- We need to end the game if the Spaceship sprite makes contact (that is, collides) with a Star.
How can we accomplish all this game logic? Put on your programmer hat, my friend—we’ll take it one step at a time.
Use the annotated Scratch code in Figure 2 as you work through the following procedures. Concentrate on why we’re coding the Star sprite the way we are. Remember that in programming, there are almost always multiple ways to accomplish the same result.

Figure 2 The Scratch code behind our Star sprite
Setting the Stage
In Figure 2, annotation A, we make the star 50 percent smaller than its original size by default, and we remove any graphic effects that may be active on the sprite from a previous run of the program. These Green Flag blocks are what I refer to as “housekeeping” or “cleanup” blocks because they reset the game environment each time the player clicks the Green Flag.
After a one-second delay (shown in annotation B), we kick off a broadcast I called StarAppear that triggers the main in-game behavior of the Star sprites. I like to add stop this script blocks wherever possible to “tie up loose ends,” as it were, with my block stacks.
Making Stars Appear
To program the stars to appear on the Stage, we’ll discuss Figure 2, annotation C. Here we set up a Forever loop that performs the following actions:
- We bump up the size of the Star by a factor of 2 and explicitly reveal the sprite on the Stage.
- We place the sprite in a random location on the Stage (recall that the Stage measures 480 pixels wide by 360 pixels high).
- We spin the star to a random angle.
In annotation D, you’ll see that I backed off the sprite from the top, bottom, left, and right borders of the Stage to prevent Stars from showing up partially off-playfield. In annotation E, we instruct Scratch to wait one second after spawning the first Star before creating a clone; this clone follows the logic of the forever loop, resulting in an ever-increasing number of stars visible on the playfield. The point here is to gradually ramp up the difficulty of the game, like any self-respecting arcade videogame does.
Handling Collision Detection
Oh, there are so many ways for handling collision detection in graphical computer programs. What we’re doing in annotation F is specifying that when the hit broadcast is received by a Star sprite (and no, we haven’t yet defined the hit broadcast—be patient!), the pop sound fires and that particular clone is removed from the playfield.
What we’ll see later is that the hit event is fired when the Spaceship’s laser beam makes contact with a Star sprite.
Of course, we recall that we add the pop sound (or any other, as your preferences dictate) by visiting the Sounds palette for the Star sprite.
Finally, check out annotation G. Here we hide all Stars if the EndGame broadcast is triggered. Again, we’ll program that particular event momentarily.
Programming the Spaceship
Now let’s turn our attention to the player’s Spaceship sprite. Our first challenge is to think about how we’ll handle the collision detection between the Spaceship’s laser beam and a Star.
Remember from the Part 1 article, we programmed the Spaceship costume to switch when the player presses the SPACE (fire) key. In the original version of this game, the laser bolt is yellow, which is the same color as our Star.
If you’ve tried to do collision detection in Scratch before, then you’ll see the problem. We’re going to program a rule such that whenever the color of the Spaceship’s laser bolt connects with the color of the Star, the hit broadcast message is raised. Of course, this isn’t going to work if both sprites are yellow!
So…the long story short is that we need to change some colors around. Look at Figure 3 to see what we need to do.

Figure 3 The Scratch Paint Editor allows us to customize a sprite’s appearance
Select the Spaceship sprite, navigate to the Costumes palette, and click the spaceship-fire costume.
Next, use the Paint Bucket and color swatch controls to recolor the laser bolt. All you have to do is click the laser bolt once, and the Paint Bucket takes care of the rest. I chose blue to present a clear color contrast between the Spaceship, Stars, and playfield background colors.
All set? Great. Now please return to the Scripts palette and we’ll code the game logic for the Spaceship from end to end. Use Figure 4 as your reference as I walk you through the admittedly complex code.
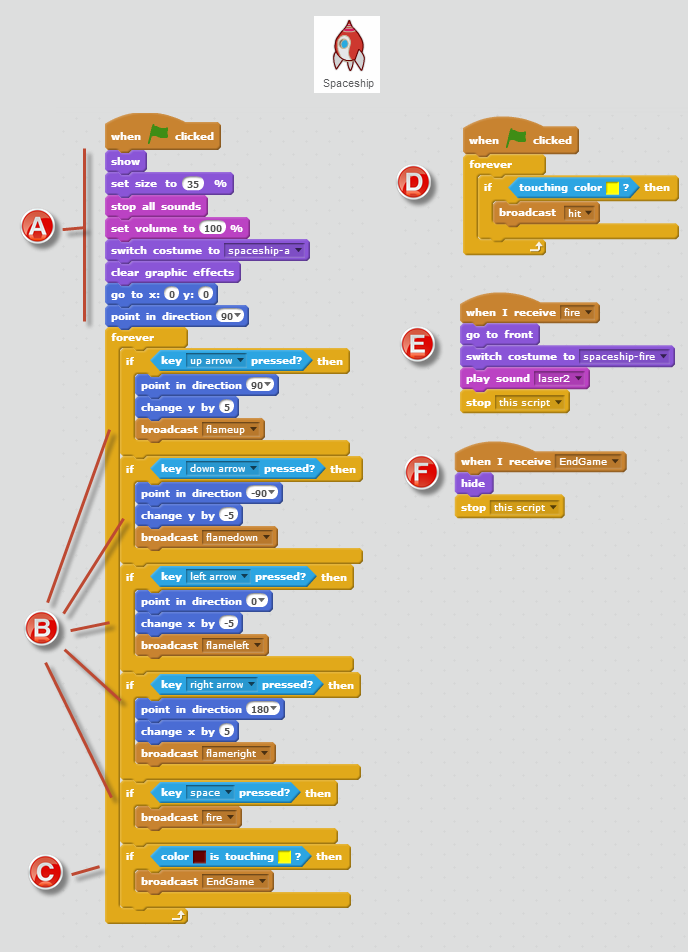
Figure 4 The Scratch code behind our Spaceship sprite
Resetting the Environment
In Figure 4 annotation A, we perform various and sundry housekeeping tasks to ensure that the Spaceship is reset to default values every time that the game is started.
We covered much of the logic in annotation B in Part 1 of this article series. The code in the Forever loop executes continuously until a game-ending event occurs, which is specifically if or when the Spaceship connects with a Star sprite.
Programming Collision Detection, Revisited
In Figure 4 annotation C, we use the color is touching block to code the collision detection between the laser/lightning bolt color we selected earlier and the Star color. This block is particularly confusing, so let me give you the step-by-step on its use:
- Click in the first color swatch in the color is touching block.
- Now click on a sample of that color. To accomplish this procedure, you’ll need to have both a star and the Spaceship on Stage simultaneously. In this case, click the border color of the Spaceship sprite; you should see the first block in the color is touching block pick up that color.
- Now perform the same two-step sequence on the second color is touching color block and the Star shape.
If we translate annotation C to what we programmers call psuedocode, then we can say that “if any part of the Spaceship’s border color touches the Star sprite color, then send out a broadcast called ‘EndGame.’”
Now have a look at annotation D. Here we specify that if any other part of the Spaceship (especially the lightning bolt) touches the Star’s yellow color, then we transmit the hit broadcast message. Recall earlier in this tutorial that when the Star receives the hit broadcast, it disappears.
We covered the code in annotation E in the previous article; here we handle the fire event that is raised when the player presses SPACE. In annotation F, we hide the sprite when we end the game.
Speaking of ending the game, I think it would be appropriate to show the player a formal “GAME OVER” screen to signal the final game state, wouldn’t you agree? Let’s take care of that now.
Customizing the Stage
Take a look at Figure 5 to see my vision for a “Game Over” screen.
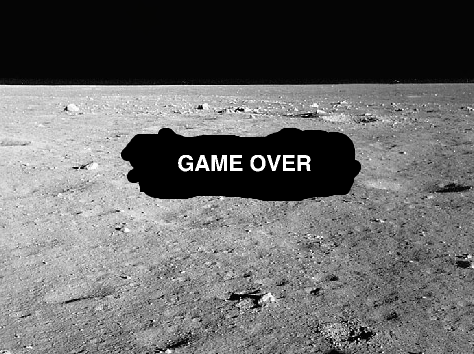
Figure 5 Our Game Over screen
The point here is that we want to graphically show the user that the game has ended. The challenge here is that we need any on-screen elements, such as the Spaceship and the Stars, to disappear.
We actually handled that by attaching hide blocks to the EndGame message, so all we really need to do is actually create the screen and define its logic.
Drawing the Game Over Screen
Click the Stage and navigate to the Backdrops palette in the Scratch Online Editor. Visit the Backdrop Library and import the moon backdrop from the Space category. Rename the backdrop gameover.
You can then use the Brush and Text tools to draw a canvas and add the words GAME OVER, as shown in Figure 6.
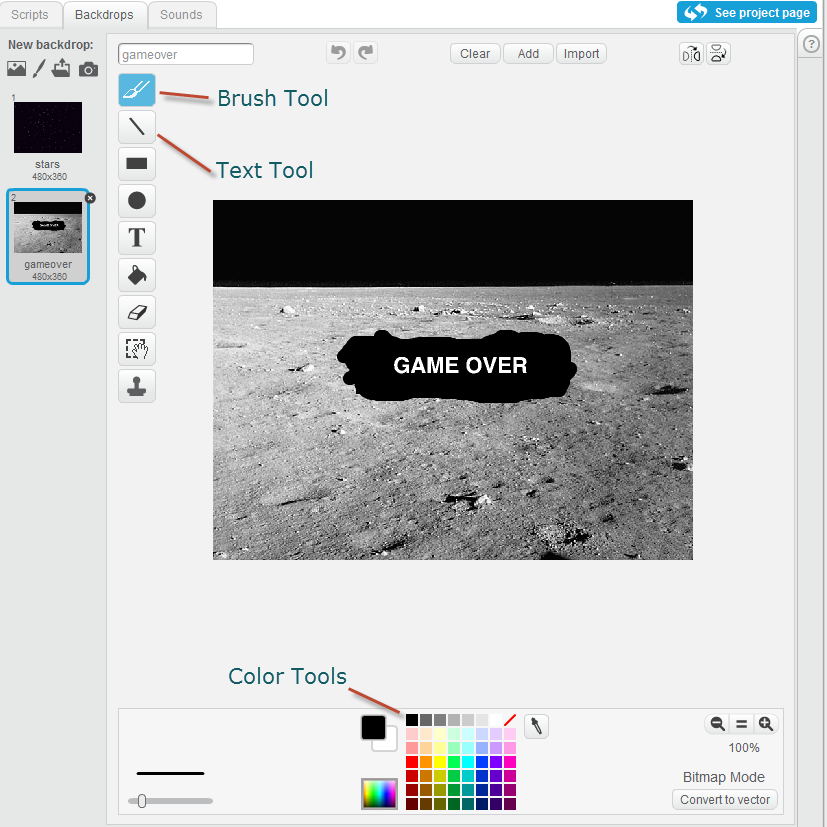
Figure 6 Crafting our Game Over screen
Programming the Game Over Screen
Finally, take a look at the annotations in Figure 7 and I’ll walk you through the last bit of code in this project.

Figure 7 The Scratch code behind the Stage
In Figure 7 annotation A, we reset the backdrop to stars every time the player clicks the Green Flag to start a new game session. In annotation B, we respond to the EndGame broadcast message by performing the following actions:
- Switching the backdrop to gameover.
- Playing the spaceripple sound (naturally, you’ll need to add the sound clip to the Stage; you should know how to do this by now).
- Wait three seconds and stop all scripts, effectively ending the game.
Next Steps
I’m sorry to use the cliché, but we truly have only scratched the surface of where we can take this simple game. You and I have reached the end of the road here, but I want to challenge you to enhance the game in at least the following ways:
- Scoring. At the moment, we have no way to track the player’s progress. Flex your Scratch programming skills by implementing a scoring system. How about the player earns 1 point for every destroyed star?
- Better Effects. Right now, the Star simply disappears when it is “hit” by the Spaceship’s lightning laser. Figure out a way to add graphical effects to the Stars such that they actually appear to explode when hit.
- Diagonal Movement. At the moment, the Spaceship is limited to movement in four directions only. Find a way to support diagonal movement; believe me, it’s possible. For example, when the player presses the UP ARROW and RIGHT ARROW keys simultaneously, the ship points northeast and travels as such.
Good luck to you, and happy Scratching!